Understanding Java Object-Oriented Programming (OOPS) with Naruto Characters
Introduction:
Java Object-Oriented Programming (OOPS) is a fundamental concept in Java programming that allows developers to structure their code efficiently and create modular and reusable software. In this unique and entertaining blog post, we’ll explore the world of Naruto and use its beloved characters to explain key concepts of Java OOPS. So, get ready to dive into the ninja realm as we uncover the secrets of OOPS with Naruto characters!
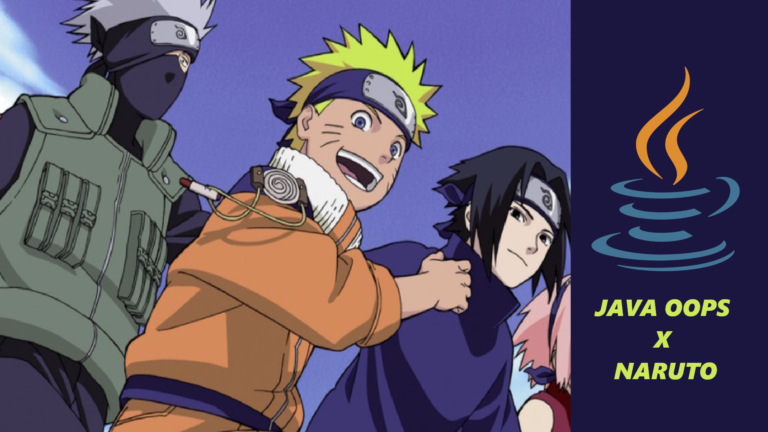
1. Encapsulation: Naruto Uzumaki – The Hidden Power within
In the Naruto universe, Naruto Uzumaki carries the essence of encapsulation. Just as Naruto hides the immense power of the Nine-Tails within himself, encapsulation in Java enables us to bundle data and methods together, protecting them from external interference. Naruto’s character perfectly exemplifies how encapsulation maintains the integrity and privacy of important attributes, only allowing controlled access through well-defined methods.
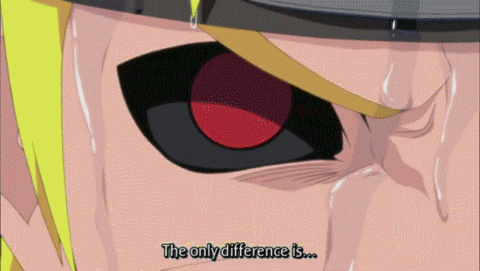
//Example Code - Encapsulation
public class NarutoUzumaki {
private String hiddenPower;
public void setHiddenPower(String power) {
this.hiddenPower = power;
}
public String getHiddenPower() {
return hiddenPower;
}
}
public class Main {
public static void main(String[] args) {
NarutoUzumaki naruto = new NarutoUzumaki();
naruto.setHiddenPower("Nine-Tails Chakra");
System.out.println(naruto.getHiddenPower()); // Output: Nine-Tails Chakra
}
}
2. Inheritance: Sasuke Uchiha – The Legacy of Sharingan
Sasuke Uchiha is known for his Sharingan, a powerful eye technique inherited from his Uchiha clan. Similarly, inheritance in Java allows us to create new classes that acquire properties and behaviors from existing classes. Sasuke’s lineage of Sharingan showcases how subclasses can inherit and extend the attributes and methods of their parent class. Just as Sasuke builds upon his clan’s abilities, Java inheritance enables code reuse and promotes hierarchical relationships.
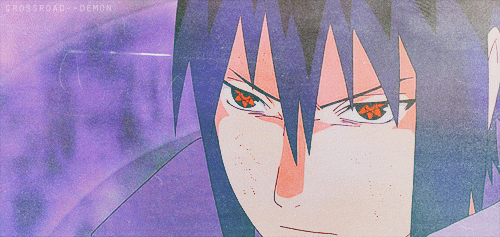
//Example Code - Inheritance
public class Uchiha {
public void useSharingan() {
System.out.println("Using Sharingan...");
}
}
public class Sasuke extends Uchiha {
public void useAmaterasu() {
System.out.println("Using Amaterasu!");
}
}
public class Main {
public static void main(String[] args) {
Sasuke sasuke = new Sasuke();
sasuke.useSharingan(); // Output: Using Sharingan...
sasuke.useAmaterasu(); // Output: Using Amaterasu!
}
}
3. Polymorphism: Kakashi Hatake – The Master of a Thousand Techniques
Kakashi Hatake, a skilled and versatile shinobi, is the epitome of polymorphism. Polymorphism in Java empowers objects to take on multiple forms, depending on the context. Like Kakashi, who effortlessly adapts to different situations with his vast array of jutsus, Java polymorphism allows objects of different classes to be treated as instances of a common superclass or interface. This flexibility enables code to be written in a more generic and adaptable manner.
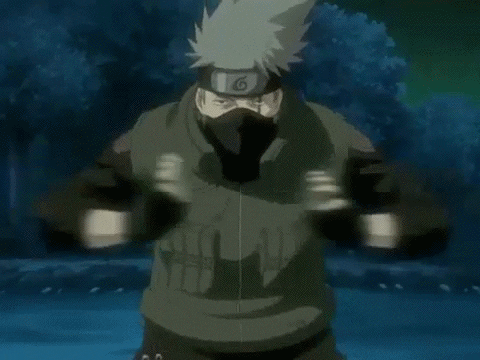
//Example Code - Polymorphism
public interface Jutsu {
void use();
}
public class FireballJutsu implements Jutsu {
@Override
public void use() {
System.out.println("Using Fireball Jutsu!");
}
}
public class LightningBlade implements Jutsu {
@Override
public void use() {
System.out.println("Using Lightning Blade!");
}
}
public class Main {
public static void main(String[] args) {
Jutsu jutsu1 = new FireballJutsu();
Jutsu jutsu2 = new LightningBlade();
jutsu1.use(); // Output: Using Fireball Jutsu!
jutsu2.use(); // Output: Using Lightning Blade!
}
}
4. Abstraction: Shikamaru Nara – The Strategic Genius
Shikamaru Nara, a strategic genius, represents the concept of abstraction in Java. Just as Shikamaru focuses on the bigger picture and hides unnecessary details, abstraction in programming helps manage complexity by providing a simplified view of objects. Abstraction allows us to define classes with essential characteristics and behaviors while hiding the underlying implementation details. It enables us to work with high-level concepts and create more manageable and modular code.

//Example Code - Abstraction
abstract class Ninja {
public abstract void performAction();
}
public class Shikamaru extends Ninja {
@Override
public void performAction() {
System.out.println("Executing Shadow Possession Jutsu!");
}
}
public class Main {
public static void main(String[] args) {
Ninja ninja = new Shikamaru();
ninja.performAction(); // Output: Executing Shadow Possession Jutsu!
}
}
Conclusion:
By exploring the world of Naruto and its dynamic characters, we’ve gained a unique perspective on Java Object-Oriented Programming (OOPS). Through the examples of Naruto, Sasuke, Kakashi, and Shikamaru, we’ve uncovered the key concepts of encapsulation, inheritance, polymorphism, and abstraction.
Remember, just as these characters master their respective abilities, understanding and applying OOPS concepts in Java will empower you to build robust, scalable, and efficient software. So, harness the ninja spirit and incorporate the power of OOPS into your Java programming journey!
Disclaimer: This blog post is purely fictional and intended for entertainment purposes only. No official endorsement or affiliation with Naruto or its characters is implied.
I hope, the article helps you find the information you want.💕
Please encourage us by rating the article and sharing your thoughts through comments.😊
Happy Coding!! 🫡
Related Article:Â How does a Java program run and what is the role of the JVM?