Marvelous Functional Programming in JavaScript
Introduction:
Welcome to the world of functional programming, where coding meets the Marvel Universe! Join The Avengers as they embark on a hilarious and action-packed adventure to explore functional programming concepts in JavaScript. Get ready to witness epic code battles and unleash the power of pure functions, immutability, higher-order functions, and more!
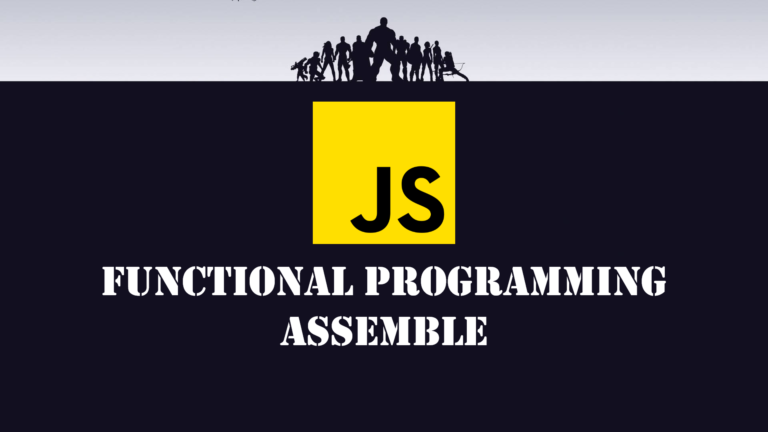
1. Pure Functions: Captain America’s Shield of Predictability
Captain America, the embodiment of righteousness, showcases pure functions that are as reliable as his iconic shield. In functional programming, pure functions always produce the same output for the same inputs and have no side effects. They rely only on their input parameters, making them predictable and easier to reason about. Just like Captain America, pure functions maintain their integrity.
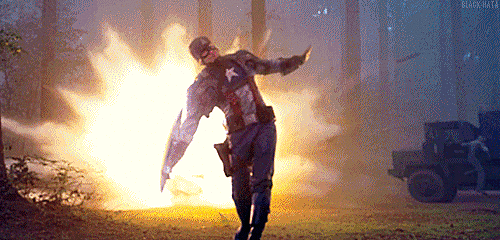
// Captain America's Pure Function
function throwShield(strength) {
return `Shield thrown with a strength of ${strength}!`;
}
console.log(throwShield(100)); // Output: Shield thrown with a strength of 100!
Here, the throwShield
function takes a strength
parameter and returns a string indicating the strength of the shield throw. Since the function does not modify any external state or rely on it, it remains pure.
2. Immutability: Thor’s Mjölnir, the Immutable Hammer
Thor, the God of Thunder, wields his mighty hammer Mjölnir, a symbol of immutability. In functional programming, data is immutable, meaning it cannot be changed after creation. Instead of modifying existing data, new data is created, leaving the original data unchanged. This ensures data integrity and prevents unintended side effects. Thor demonstrates immutability through his handling of Mjölnir.
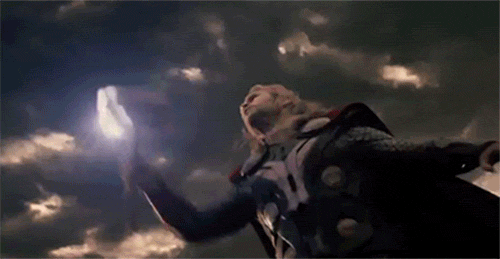
// Thor's Immutable Data
const originalHammer = { name: 'Mjölnir', power: 'Storm-summoning' };
const newHammer = { ...originalHammer, owner: 'Thor' };
console.log(newHammer); // Output: { name: 'Mjölnir', power: 'Storm-summoning', owner: 'Thor' }
Here, the originalHammer
object remains untouched, and a new object newHammer
is created with an additional owner
property using the spread syntax (...
). This way, the original data is preserved while incorporating new changes.
3. Higher-Order Functions: Tony Stark’s Iron-Suit Upgrades
Tony Stark, the brilliant inventor, exemplifies higher-order functions as he constantly upgrades his Iron Man suits. Higher-order functions can accept other functions as arguments or return functions. They enable code abstraction, reusability, and composability, empowering developers to level up their coding game. Let’s see how Tony writes his code using higher-order functions.
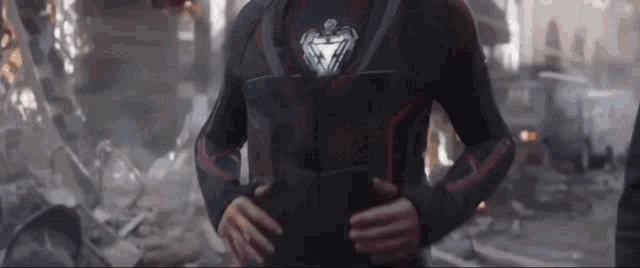
// Tony's Higher-Order Function
function buildSuit(upgradeFunction) {
console.log('Assembling Iron Man suit...');
upgradeFunction();
}
function mark50() {
console.log('Installing Mark 50 upgrade...');
}
buildSuit(mark50); // Output: Assembling Iron Man suit... Installing Mark 50 upgrade...
In this example, the buildSuit
function is a higher-order function that takes another function upgradeFunction
as an argument. It then executes the upgradeFunction
within its own logic, allowing for dynamic behavior and extensibility.
4. Function Composition: Black Widow’s Stealthy Moves
Black Widow, the master spy and assassin, demonstrates the power of function composition with her stealthy moves. Function composition involves combining multiple functions to create a new function. By chaining functions together, developers can create powerful and reusable operations. Let’s observe Black Widow perform function composition in code.
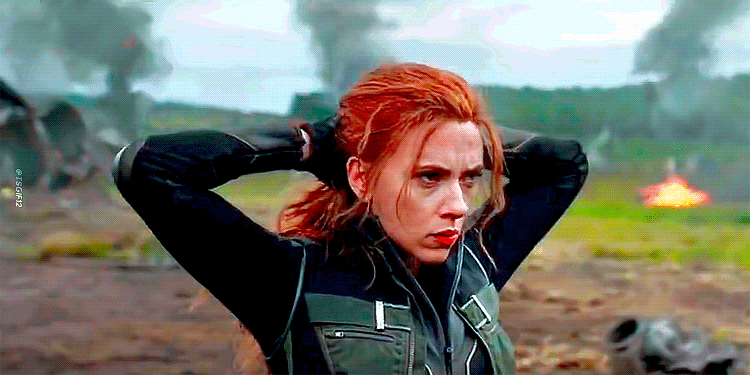
// Black Widow's Function Composition
const attack = (target) => `Attacking ${target}!`;
const sneak = (action) => `Sneaking: ${action}`;
const stealthAttack = (target) => sneak(attack(target));
console.log(stealthAttack('Loki')); // Output: Sneaking: Attacking Loki!
In this example, the stealthAttack
function is composed by chaining the sneak
and attack
functions. It first applies the attack
function to the target
and then passes the result to the sneak
function. This composition creates a new function that executes both actions, representing Black Widow’s stealthy attack.
5. Recursion: Hulk’s Endless Rampage
Hulk, the uncontrollable force of nature, embodies recursion in functional programming. Recursion involves a function calling itself to solve a problem, allowing for elegant and compact code. Just like Hulk’s relentless rampage, recursion continues until a base condition is met. Let’s witness Hulk’s fury through a recursive function.

// Hulk's Recursive Rampage
function hulkRampage(smashCount) {
if (smashCount === 0) {
return "Hulk is finally calm!";
} else {
console.log(`Hulk SMASH! (${smashCount} smashes remaining)`);
return hulkRampage(smashCount - 1);
}
}
console.log(hulkRampage(3));
// Output:
// Hulk SMASH! (3 smashes remaining)
// Hulk SMASH! (2 smashes remaining)
// Hulk SMASH! (1 smashes remaining)
// Hulk is finally calm!
hulkRampage
function recursively calls itself, decrementing the smashCount
until it reaches 0. With each iteration, it displays the remaining smashes until Hulk finally calms down.Conclusion:
🚀 Unleash Your Inner Superhero! Explore Functional Programming with The Avengers! 💻
🌟 Rate and Share Your Thoughts! 😊
🦸♂️ Happy Coding! 🔥
Explaining in easy way to understand! good work!